Proxies
To learn how to generate an OData client, follow this tutorial. This article assumes you have generated a client for the Business Partner service from SAP S/4HANA Cloud.
Making a First API Call
Once you have generated a client, this is how you make your first API call.
businessPartnerApi
.requestBuilder()
.getAll()
.filter(businessPartnerApi.schema.BUSINESS_PARTNER_CATEGORY.equals('1'))
.top(5)
.execute(yourDestination);
What happens in the background for this call:
- Destination fetching
- Handling of ETag
- Handling of CSRF token
- Serializing and deserializing the request
This document focuses on the proxy part and explains how proxies are handled by the SAP Cloud SDK.
What Kind of Proxies are There?
It is important to have an overview of the different proxy types. There are three possible types of proxies:
- A local proxy running on the cloud platform that connects to SAP S/4HANA On-Premise systems. The proxy information like host and port are provided by the connectivity service. This proxy will be referred to as connectivity proxy.
- A proxy running in some landscape that makes requests to the internet. This proxy will be referred to as web proxy.
- A proxy which is used when your destination represents a tunnel that's created via the Private Link Service.
This proxy will be referred to as
PrivateLink
proxy.
Manual Configuration
In the execute()
you can either give an object containing the destination name or you can configure the full destination manually.
If you provide the destination name the SAP Cloud SDK will try to look up as described here.
In productive use, the manual configuration will not be useful, but for testing, it might be valuable.
The destination object contains a field proxyConfiguration
in which you can configure a proxy.
{
url: "https://MyDestinationURL.com"
proxyConfiguration: {
host: "my.proxy.host.com",
port: 123,
protocol: "http",
headers: {
Proxy-Authorization: 'yourAuthHeader could be basic or some bearer token',
SAP-Connectivity-Authentication: ''
}
}
}
The SAP-Connectivity-Authentication
field contains the JWT
issued for the user on application login.
This is mandatory if principal propagation is used i.e. identity propagation from the cloud application to the SAP S/4HANA system.
The Automatic Flow
For productive use, you do not want to include a specific proxy configuration in your code.
Here you should let the SAP Cloud SDK handle everything.
Hence, you will provide in the .execute()
method only the destination name.
This will trigger the destination lookup as described here.
The result of the lookup is a destination object which contains a property called ProxyType
.
This property can have three values: Internet
, OnPremise
, and PrivateLink
:
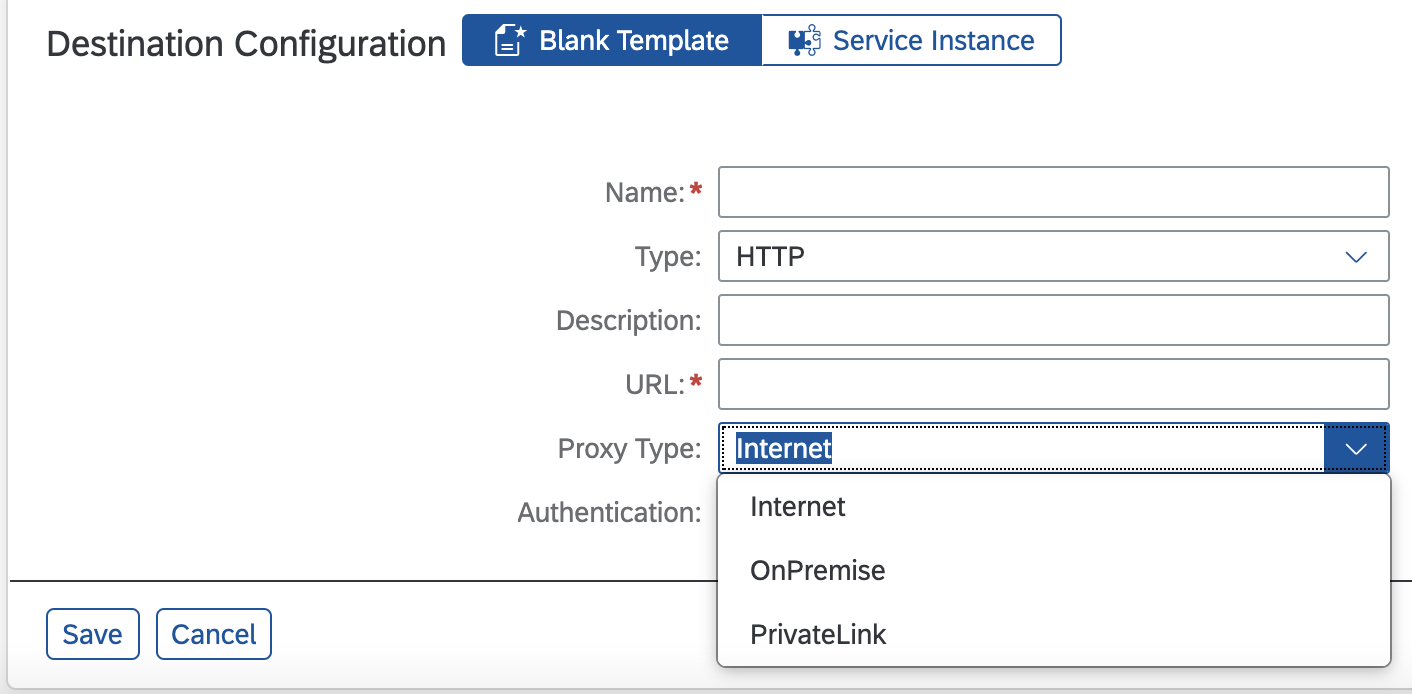
If OnPremise
is selected, it is assumed that this destination points to an SAP S/4HANA On-Premise system.
In this case, you need the connectivity proxy.
The SAP Cloud SDK will request the proxy host and port from the connectivity service and make a request using this proxy.
From there the request will pass via the SAP Cloud Connector to the SAP S/4HANA On-Premise system.
If Internet
is selected it is assumed that this destination points to something on the Internet and usually no proxy is needed.
However, if a proxy is needed to reach this destination it is configured in the following way.
The SAP Cloud SDK considers the environment variables HTTP_PROXY
and HTTPS_PROXY
.
The variable HTTP_PROXY
is used for destinations using http://
in their URL and HTTPS_PROXY
for the ones using https://
.
HTTPS is the default protocol if the protocol is not set in the URL field of the destination.
The value of the two proxy variables is just a simple string following this pattern:
<protocol>://<user>:<password>?@<host>:<port>
The user and password are optional and if they are left out no authentication header is added.
The protocol is also optional and the default value is HTTP since most proxies do not use an encryption layer for communication.
The default for the port is 80
for HTTP and 443
for HTTPS.
Example of valid values would be:
http://John:SecurePassword@some.host.com:1234
https://some.host.com:1234 -> will use 443 as default
some.host.com:1234 -> will use http as default
some.host.com -> will use http and 80 as default
If you use any special character in your username or password you need to encode them using percent encoding
Since environment variables are a global setting, it might become necessary to exclude some destinations from using the proxy.
This is possible using the NO_PROXY
environment variable.
The NO_PROXY
variable contains a list of comma-separated URLs for which no proxy is used.
Wild cards like *
are supported in the list.
If you select PrivateLink
, the flow is the same as for proxy type Internet
.
A Word on the Used Libraries and Implementation Details
The SAP Cloud SDK uses axios to execute HTTP requests. In principle axios should support web proxies based on the environment variables. However, the request URL using a proxy was not correctly constructed and an entry point for the connectivity proxy was anyhow necessary. Hence, the SAP Cloud SDK switches off the built-in proxy handling by axios completely by using the following axios request configuration.
{
"proxy": false
}
If a proxy becomes necessary or is configured by the discussed environment variables the SAP Cloud SDK builds directly the http-agent
or https-agent
:
{
"proxy": false,
"httpAgent": httpProxAgent || new http.Agent(),
"httpsAgent": httpsProxyAgent || new https.Agent()
}
This adds them to the axios configuration. The agents contain the proxy configuration. The standard http and https agents are used.